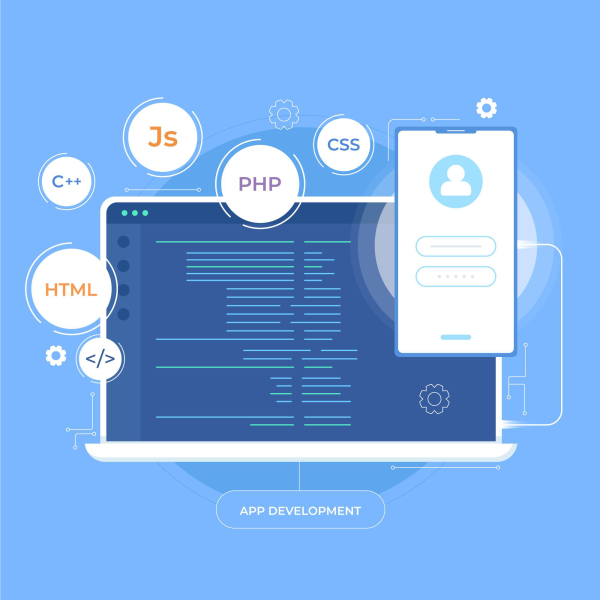
How To Add A Copy Button Inside A Pre Tag
Updated Saturday, May 4, 2024, 7 AM
When you have code blocks on a website, it can be helpful to add a "Copy" button so that visitors can easily copy the code from the pre tags to their clipboard. In this guide, we'll show you how to add a "Copy" button to all `pre` tags using HTML and JavaScript.
1. Set Up Your HTML
First, make sure you have some code blocks in your HTML using `pre` tags. For example:
<pre>
<code>console.log("Hello, world!");</code>
</pre>
This code block shows a simple JavaScript console log statement. We want to add a "Copy" button next to this code block.
2. Add JavaScript
We will write a JavaScript function that will:
- Add a "Copy" button next to each `pre` tag.
- Copy the code inside the `pre` tag when the button is clicked.
Add the following JavaScript code to your HTML file:
<script>
document.addEventListener("DOMContentLoaded", function() {
// Find all pre tags on the page
var preTags = document.querySelectorAll("pre");
// Loop through each pre tag
preTags.forEach(function(pre) {
// Create a button element
var button = document.createElement("button");
button.innerText = "Copy";
// Set up a click event listener on the button
button.addEventListener("click", function() {
// Get the code inside the pre tag
var code = pre.innerText;
// Create a temporary textarea element to copy the code
var tempTextArea = document.createElement("textarea");
tempTextArea.value = code;
document.body.appendChild(tempTextArea);
// Select and copy the text
tempTextArea.select();
document.execCommand("copy");
// Remove the temporary textarea
document.body.removeChild(tempTextArea);
// Alert the user that the code has been copied
alert("Code copied to clipboard!");
});
// Insert the button before the pre tag
pre.parentNode.insertBefore(button, pre);
});
});
</script>
This script waits for the web page to load, then it finds all `pre` tags on the page. For each `pre` tag, it creates a "Copy" button and adds it before the code block. When you click the button, the script copies the code inside the `pre` tag to the clipboard and alerts the user.
3. Try It Out
Now that you have the JavaScript code in your HTML file, open the file in a web browser to see the "Copy" buttons next to your code blocks. Try clicking the "Copy" button to see how it works!
4. Conclusion
Adding a "Copy" button to code blocks is a great way to make your website more user-friendly. By following this guide, you can easily add "Copy" buttons to all `pre` tags on your web page using HTML and JavaScript.
No comments yet