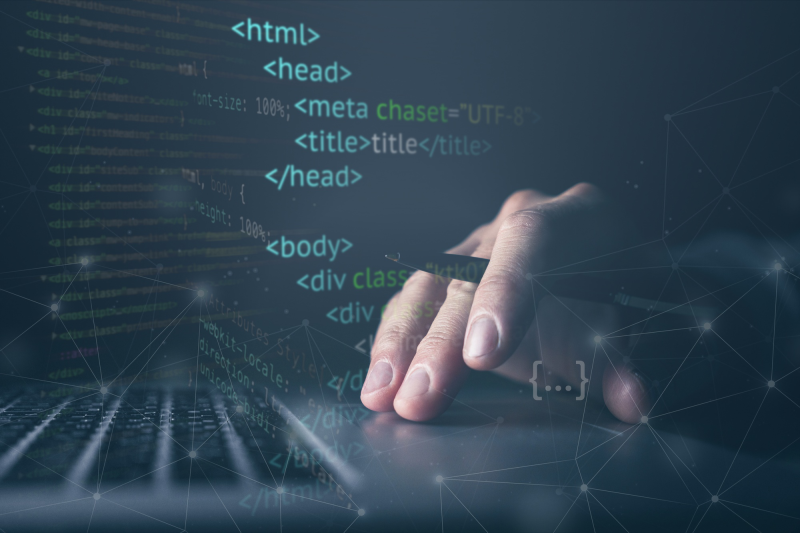
What is PHP? A Beginner's Guide to Understanding PHP
Updated Saturday, May 4, 2024, 12 PM
PHP is a server side special language that helps make websites more interactive and fun! It can create dynamic pages that change depending on what the user does. Let's learn about PHP and how it can be used to make websites come to life.
1. What is PHP?
PHP stands for "PHP: Hypertext Preprocessor." It's a language that lets you write code to control what happens on a web page. For example, PHP can do math, check information, and even store data in a database.
When someone visits a website, the web browser sends a request to the web server. If the web page contains PHP code, the server will run the PHP code and send the final HTML to the browser for the user to see. This makes the web page dynamic and interactive!
2. Writing PHP Code
To write PHP code, you use special tags to tell the server where the PHP code starts and ends. The PHP code goes between these tags:
<?php
// This is a comment. The server will ignore it.
echo "Hello, world!"; // This code displays the text "Hello, world!"
?>
3. PHP Basics
Here are some basic things you can do with PHP:
Display Text
Use the echo
function to display text on the web page:
echo "Hello, world!";
Variables
In PHP, you can use variables to store information, such as numbers or text. To create a variable, use a dollar sign ($) followed by the variable name:
$name = "Alice";
$age = 10;
echo "My name is $name and I am $age years old.";
This code creates two variables and displays the text "My name is Alice and I am 10 years old."
If Statements
Use an if statement to run code only if a certain condition is true:
$number = 10;
if ($number > 5) {
echo "The number is greater than 5!";
} else {
echo "The number is 5 or less.";
}
This code checks if the variable $number
is greater than 5. If it is, it displays "The number is greater than 5!" Otherwise, it displays "The number is 5 or less."
4. Fun Things to Do with PHP
PHP can do many exciting things on a web page!
Display the Date and Time
PHP can show the current date and time:
echo "Today's date is " . date("Y-m-d") . " and the current time is " . date("H:i:s") . ".";
This code uses the date()
function to display the current date and time.
Get User Input
PHP can also get information from the user through forms on a web page:
<form method="post">
<label for="name">Enter your name:</label>
<input type="text" id="name" name="name">
<input type="submit" value="Submit">
</form>
This example creates a form that asks the user for their name. When the user submits the form, PHP displays a greeting message using the name they entered.
Loop Through Data
PHP can use loops to go through a list of data and do something with each item:
$colors = array("red", "blue", "green");
foreach ($colors as $color) {
echo "The color is $color.
";
}
This code creates an array of colors and uses a loop to display each color.
5. Conclusion
PHP is a powerful language that helps make websites more interactive and dynamic. By learning PHP, you can create amazing web pages that respond to user input, display data, and much more. Have fun exploring the world of PHP!
No comments yet